In mobile app development, writing clean, scalable, and testable code isn’t a luxury—it’s a necessity. As your app grows, tightly coupled components can make even small updates feel like untangling a web. This is where Dependency Injection (DI) shines.
Dependency Injection is a design pattern that allows objects to receive their dependencies from an external source rather than creating them internally. This might sound abstract, but in real-world development, it’s one of the most practical patterns you can use.
Let’s start with a simple example. Imagine you have a UserManager
class that handles login and registration. This class relies on a NetworkService
.
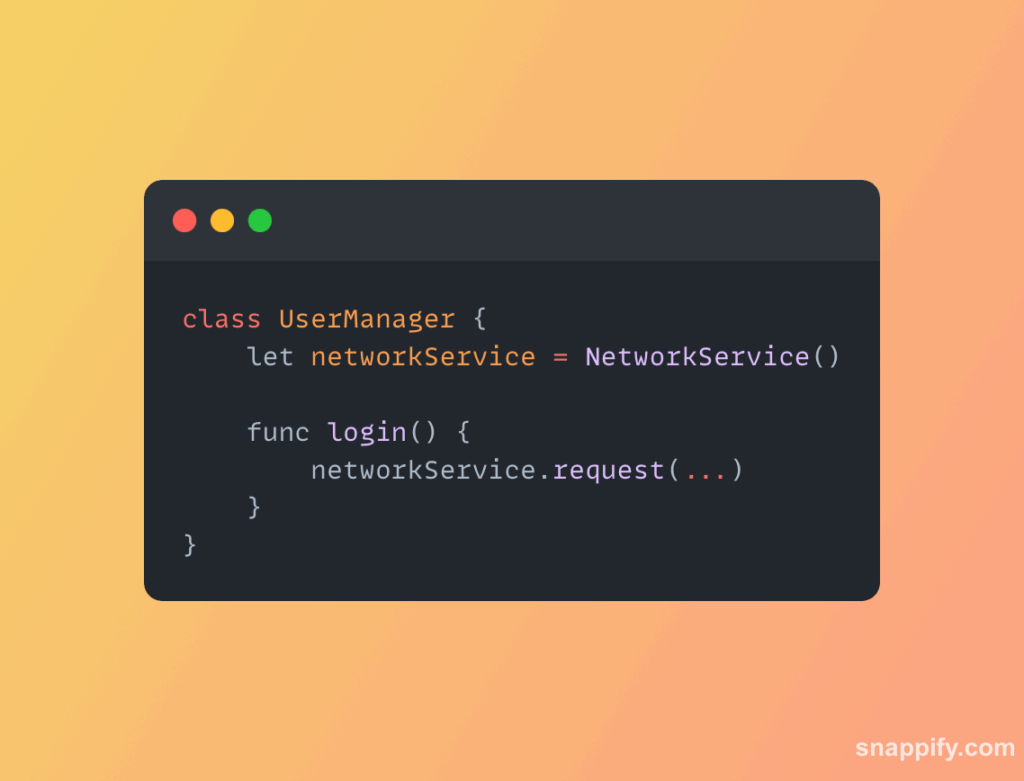
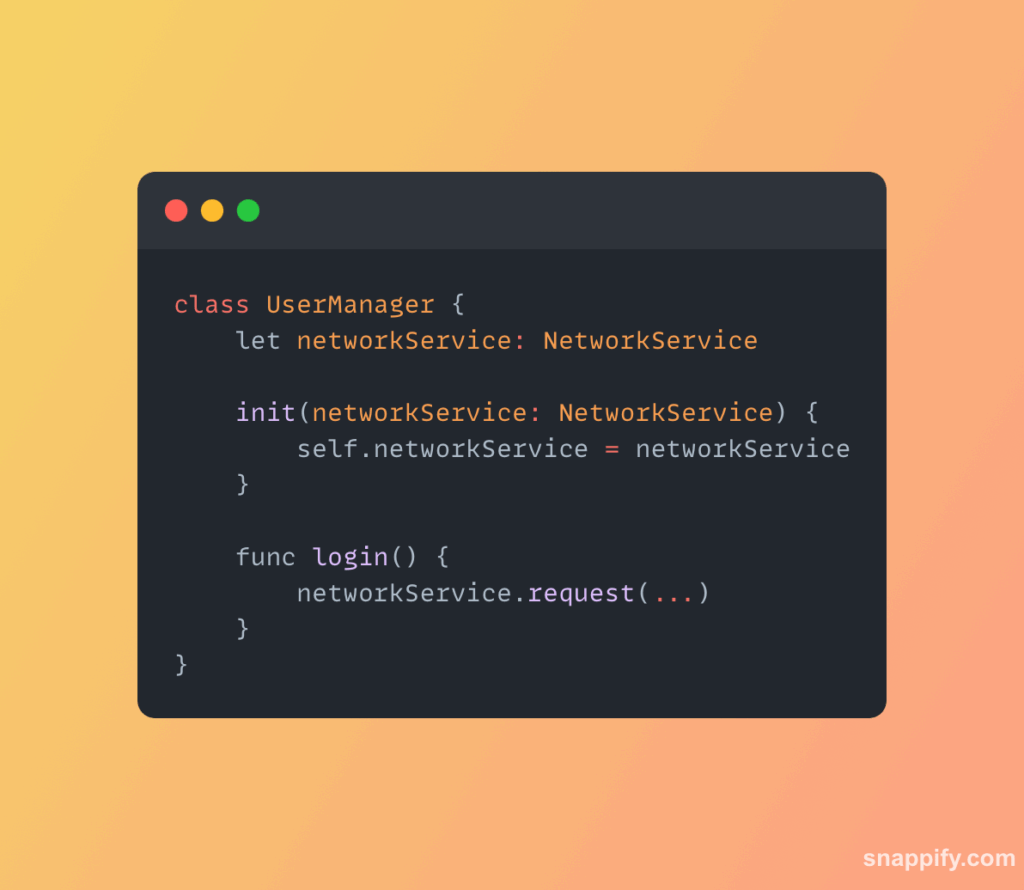
Now UserManager
doesn’t need to know how NetworkService
is created—it just uses it. You can easily swap in a mock NetworkService
for testing.
🛠️ Benefits of Dependency Injection
Improved Testability
You can inject mock implementations during unit testing without altering the production code. For example, inject a MockNetworkService
that simulates API responses without network calls.
Better Code Reusability
Components don’t rely on specific implementations. This makes classes more flexible and interchangeable.
Easier Maintenance
Changing one class has less chance of affecting others. This promotes low coupling and high cohesion.
Simplified Refactoring
Need to switch from Firebase to Supabase? Just swap the implementation injected—no rewrites required in business logic.
Cleaner Architecture
DI supports principles like SOLID, especially the Dependency Inversion Principle. It makes your codebase more modular and scalable.
Another key benefit of Dependency Injection in mobile development is the ability to build modular, scalable codebases. By decoupling components from their dependencies, teams can iterate faster, test components in isolation, and plug in new implementations without massive refactoring. This is particularly powerful when building enterprise-grade mobile applications where multiple teams may work on different modules.
For instance, consider a shopping app with features like cart, checkout, payments, and reviews. Each feature module can define its own interfaces and request dependencies via constructor injection. A DI container (like Koin or Hilt) takes care of instantiating and providing the correct implementation. This promotes separation of concerns, aligns with mobile architecture best practices, and enables feature toggling or A/B testing by swapping implementations dynamically.
Many modern mobile app teams use a dependency injection framework to manage object graphs. These frameworks not only reduce boilerplate but also enforce architectural patterns like Clean Architecture or MVVM. For example, using Hilt in Android or Resolver in Swift ensures that your ViewModel doesn’t need to know how to create its repository—it just asks for it.
Additionally, when testing components like API clients or local databases, dependency injection allows injecting mock or fake implementations. This leads to faster unit testing, improved CI/CD pipelines, and better test coverage overall.
Another emerging use case is in cross-platform development. Frameworks like Kotlin Multiplatform Mobile (KMM) or Flutter allow using DI patterns in shared logic. This means developers can write shared business logic once and inject platform-specific implementations, boosting productivity.
Finally, integrating DI early in a mobile project helps avoid the tech debt of tightly coupled systems. It’s an investment that pays off with every new feature, test, or refactor.
📱 DI in Mobile Frameworks
iOS (Swift / SwiftUI)
You can manually perform DI via initializers or use libraries like Swinject or Resolver to manage object lifecycles and dependencies.
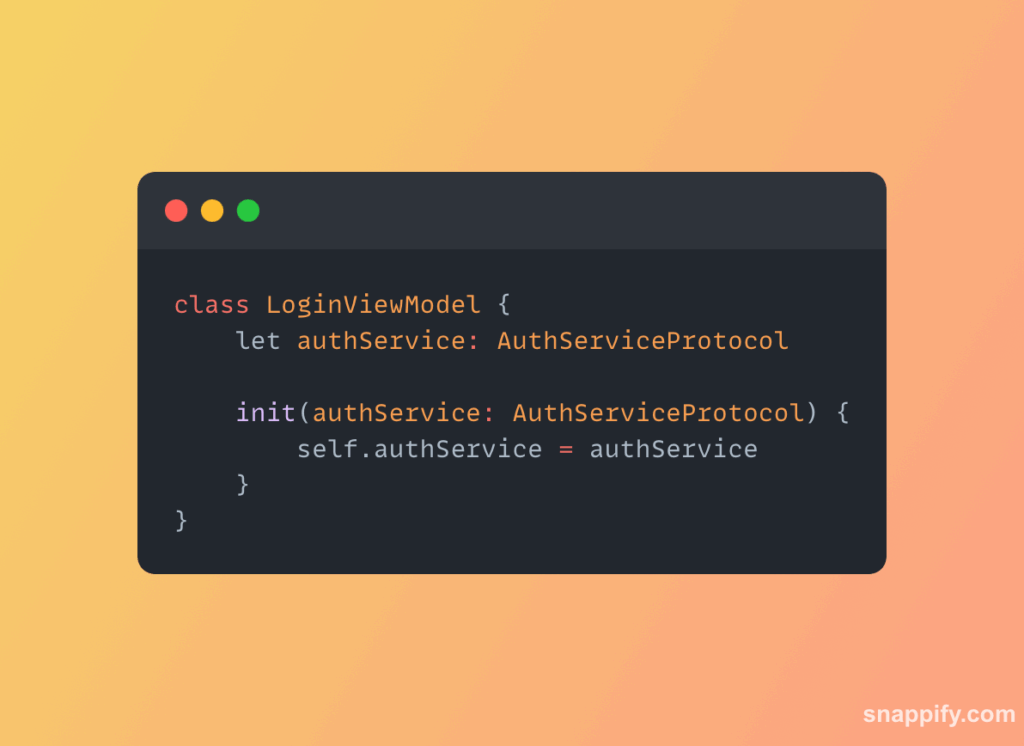
Android (Kotlin / Jetpack Compose)
Kotlin offers native support for DI using Hilt or Koin.
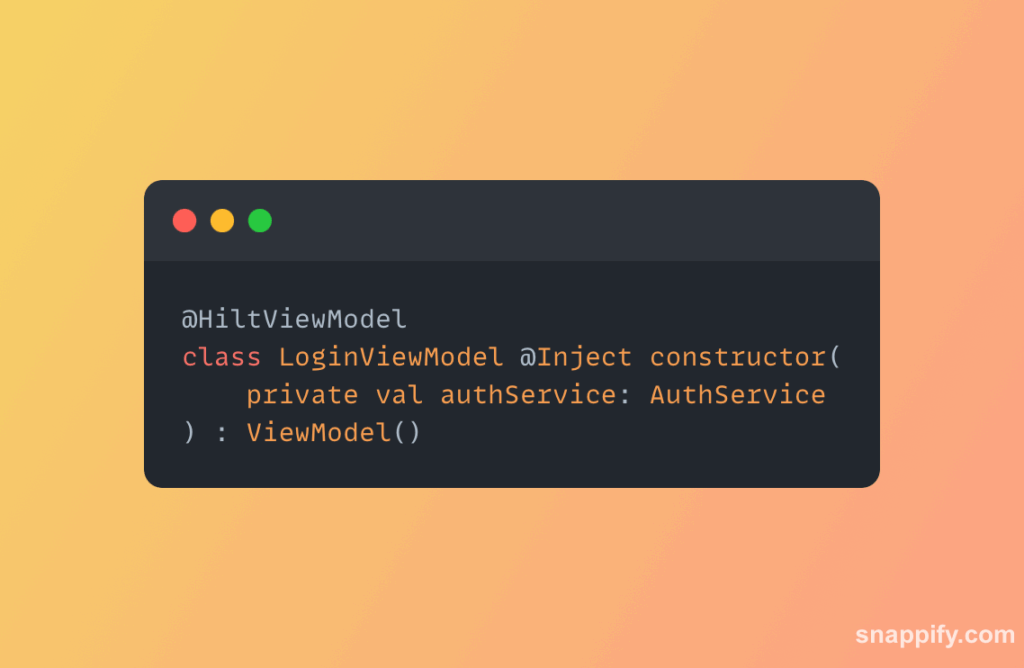
📚 Case Study: Spotify
Spotify’s mobile architecture is a great example of Dependency Injection at scale. Their apps are broken into hundreds of modules and services. DI allows Spotify’s developers to:
-
Inject only the modules needed for each feature.
-
Run feature-specific tests without starting the full app.
-
Swap backend services during A/B testing.
By decoupling services using DI, Spotify achieved faster deployments and greater modularity, leading to smoother user experiences.
🧪 Mocking Example for Testing
Let’s say you have a WeatherService
that pulls weather data from an API.
In your test, you just inject MockWeatherService
. No API needed!
🏁 Conclusion
Dependency Injection is not just for big enterprise apps—solo developers and small teams benefit just as much. The pattern keeps your code loosely coupled, easily testable, and highly scalable. Whether you’re building a simple todo app or an enterprise banking app, DI is an investment in clean architecture and long-term sustainability.